How to Debug Node.js Async/Await Memory Leaks Effectively
Memory leaks in Node.js applications can be a significant challenge, particularly when using async/await for handling asynchronous operations. These leaks can lead to increased memory consumption and eventually crash the application. Understanding how to effectively debug these issues is crucial for maintaining the performance and reliability of Node.js applications. This article will explore practical strategies and tools to identify and resolve memory leaks in Node.js applications that utilize async/await.
Understanding Memory Leaks in Node.js
Memory leaks occur when an application retains references to objects that are no longer needed, preventing the garbage collector from reclaiming that memory. In Node.js, this can happen for various reasons, such as closures, event listeners, or unhandled promises. Async/await, while simplifying asynchronous code, can inadvertently contribute to memory leaks if not handled correctly.
Common Causes of Memory Leaks
Several common patterns can lead to memory leaks in Node.js applications. For instance, if a function creates a closure that references large objects, those objects may persist longer than necessary. Similarly, if event listeners are not properly removed, they can hold references to objects that should be garbage collected.
Another common issue arises from unhandled promise rejections. If a promise is rejected and not caught, the resources associated with that promise may remain allocated, leading to memory leaks over time.
Moreover, global variables can also contribute to memory leaks. When objects are assigned to global variables, they remain in memory for the lifetime of the application, even if they are no longer needed. This can lead to significant memory consumption, especially in long-running applications. It is crucial to be mindful of variable scope and to clean up any references that are no longer necessary.
Identifying Memory Leaks
To effectively debug memory leaks, it’s essential first to identify their presence. Monitoring memory usage over time can provide insights into whether an application is experiencing a leak. Tools like Node.js's built-in `process.memoryUsage()` method can help track memory consumption, revealing trends that indicate potential leaks.
Additionally, using profiling tools can help visualize memory usage and identify problematic areas in the code. Tools such as Chrome DevTools or Node.js's built-in inspector can provide snapshots of memory usage, allowing developers to pinpoint where leaks may be occurring. By taking heap snapshots at different intervals, developers can compare memory allocations and identify objects that are not being released as expected.
Another effective strategy for identifying memory leaks is to implement logging around critical parts of the application. By logging the creation and destruction of objects, developers can track the lifecycle of objects and determine if they are being properly cleaned up. This practice not only aids in identifying leaks but also enhances overall application performance by providing insights into memory usage patterns during runtime.
Using Profiling Tools
Profiling tools are invaluable when it comes to diagnosing memory leaks in Node.js applications. They allow developers to analyze memory usage in real-time and identify which parts of the application are consuming excessive memory.

Chrome DevTools
Chrome DevTools can be used to debug Node.js applications by connecting to the Node.js process. By launching the application with the `--inspect` flag, developers can open the DevTools interface in Chrome and access various profiling features. The "Memory" tab allows for heap snapshots, which can be compared over time to identify objects that are not being garbage collected.
Heap snapshots provide a detailed overview of memory allocation, showing which objects are being retained and how much memory they consume. By analyzing these snapshots, developers can trace back to the code that is causing the leaks. Additionally, the "Timeline" feature in DevTools can help visualize memory usage over time, allowing developers to see how memory consumption fluctuates with different operations. This can be particularly useful for identifying patterns in memory usage that correlate with specific user actions or application events.
Node.js Inspector
The Node.js Inspector is another powerful tool that provides a debugging interface for Node.js applications. Similar to Chrome DevTools, it allows developers to take heap snapshots and analyze memory usage. The inspector can be launched using the command `node --inspect-brk app.js`, which pauses the execution of the application until a debugger is attached.
Once connected, developers can navigate through the code, set breakpoints, and inspect variables. This level of control is beneficial for identifying potential leaks in async/await code, as it allows for a detailed examination of the call stack and the state of the application at various points in time. Moreover, the Node.js Inspector supports the use of the "Profiler" tab, which can record CPU usage and help pinpoint performance bottlenecks in addition to memory issues. By analyzing the CPU profiles, developers can gain insights into which functions are consuming the most processing power, enabling them to optimize their code further and improve overall application performance.
Best Practices to Prevent Memory Leaks
While debugging memory leaks is essential, preventing them from occurring in the first place is even more critical. Adopting best practices can significantly reduce the likelihood of leaks in Node.js applications.
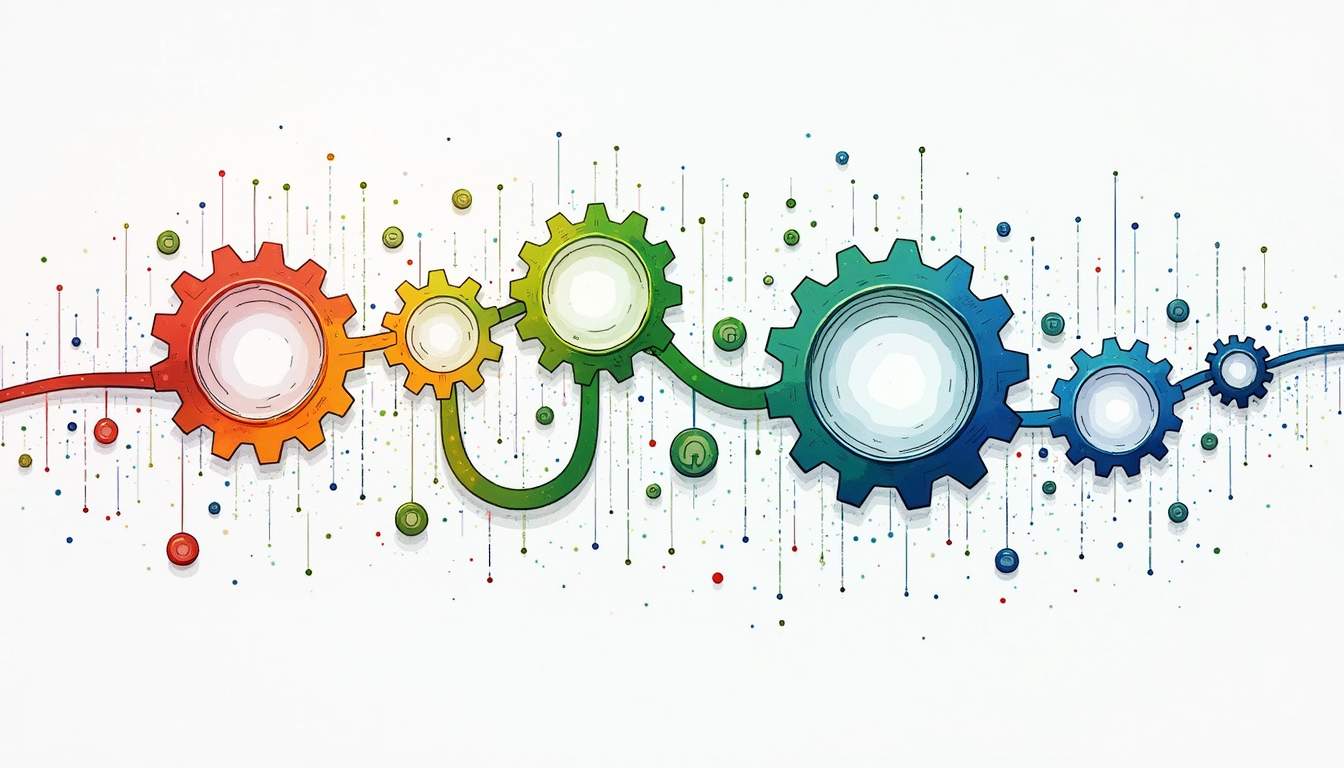
Properly Handle Promises
One of the most effective ways to prevent memory leaks is to ensure that all promises are handled correctly. This includes using `try/catch` blocks with async/await to catch any potential errors. Unhandled promise rejections can lead to retained memory, so it’s crucial to manage promise states effectively.
Additionally, using libraries that provide better promise management can help. For instance, libraries like `Bluebird` offer features such as cancellation and better error handling, which can mitigate the risk of memory leaks. By leveraging these tools, developers can create more robust applications that gracefully handle asynchronous operations, thereby reducing the chances of memory being inadvertently held onto due to unresolved promises.
Clean Up Event Listeners
Event listeners can be a source of memory leaks if they are not properly removed when no longer needed. In Node.js, it’s essential to detach listeners when they are no longer relevant, especially in long-running applications. Using named functions for event listeners can simplify the process of removing them, as anonymous functions cannot be easily detached.
Moreover, utilizing the `once` method for listeners that only need to be triggered once can help prevent unintended memory retention, as these listeners are automatically removed after their first invocation. It's also beneficial to implement a systematic approach to managing event listeners, such as keeping track of them in a centralized registry. This allows developers to audit and clean up listeners more efficiently, ensuring that the application remains responsive and free of memory bloat over time.
Furthermore, developers should be vigilant about the lifecycle of their objects and the events they are tied to. For example, when working with UI components, it’s important to remove event listeners when components are destroyed or unmounted. This practice not only helps in preventing memory leaks but also enhances the application's performance by ensuring that unnecessary references are cleared, allowing the garbage collector to reclaim memory effectively.
Debugging Async/Await Memory Leaks
Debugging memory leaks specifically within async/await code can be challenging due to the nature of asynchronous operations. However, there are strategies that can help streamline the process.
Isolate Async Functions
When debugging async functions, isolating them can provide clarity. By breaking down complex async functions into smaller, more manageable pieces, developers can more easily identify which part of the code is causing memory issues. This isolation allows for targeted testing and profiling, making it easier to spot leaks.
Additionally, using logging within async functions can help track the flow of execution and identify where memory may be accumulating. By logging the entry and exit points of functions, developers can correlate memory usage with specific operations.
Monitor Long-Running Operations
Long-running asynchronous operations can be particularly prone to memory leaks. Monitoring these operations is crucial, as they may hold references to large objects or data structures that are not released. Implementing timeouts or limiting the duration of these operations can help mitigate the risk of leaks.
Using tools like `async_hooks` can also provide insights into the lifecycle of asynchronous resources, allowing developers to track when resources are created and destroyed. This can be particularly useful for identifying leaks in complex async flows.
Case Study: Debugging a Memory Leak
To illustrate the debugging process, consider a hypothetical Node.js application that fetches data from an external API using async/await. Over time, the application experiences increased memory usage, leading to performance degradation.

Step 1: Identify the Leak
The first step in addressing the issue involves monitoring memory usage. By utilizing `process.memoryUsage()`, developers notice a steady increase in memory consumption as the application runs. This observation prompts a closer examination of the async functions responsible for data fetching.
Using Chrome DevTools, the developer takes heap snapshots at various intervals. Comparing these snapshots reveals that a significant number of objects related to the API responses are being retained in memory, indicating a potential leak.
Step 2: Analyze the Code
Upon reviewing the code, the developer discovers that the API responses are being stored in a global variable for caching purposes. However, the cache is never cleared, leading to an accumulation of data over time. This design flaw is identified as the root cause of the memory leak.
To resolve the issue, the developer implements a cache expiration strategy, ensuring that old entries are removed after a certain period. This adjustment significantly reduces memory consumption and prevents future leaks.
Conclusion
Debugging memory leaks in Node.js applications, particularly those that utilize async/await, can be a complex but manageable task. By understanding the common causes of memory leaks, utilizing profiling tools, and adopting best practices, developers can effectively identify and resolve these issues.
As applications grow in complexity, ongoing monitoring and proactive debugging become essential. By implementing the strategies discussed in this article, developers can maintain optimal performance and ensure that their Node.js applications remain reliable and efficient.
Streamline Your Development with Engine Labs
As you tackle the complexities of memory leaks in your Node.js applications, consider the power of Engine to enhance your development workflow. Engine Labs is at the forefront of AI-driven software engineering, offering a revolutionary solution that integrates with your favorite project management tools to automate up to half of your tickets. With Engine, you can minimize the time spent on mundane tasks and focus on resolving critical issues like memory leaks, pushing your projects to completion with unprecedented speed. Ready to transform your development process and bid farewell to those persistent backlogs? Get Started with Engine Labs today and propel your team into a new era of efficiency.