Mastering TypeScript Generics Constraints in React Hooks
TypeScript has gained immense popularity among developers, especially when working with React. Its ability to provide type safety and enhance code quality makes it a preferred choice for many projects. One of the most powerful features of TypeScript is its support for generics, which allows developers to create reusable components and functions. However, when combined with React hooks, generics can become complex, especially when constraints are involved. This article delves into mastering TypeScript generics constraints in the context of React hooks, providing insights and practical examples to help developers navigate this powerful feature.
Understanding TypeScript Generics
Before diving into generics constraints, it's crucial to grasp what generics are and how they function in TypeScript. Generics allow developers to create components or functions that can work with any data type while maintaining type safety.
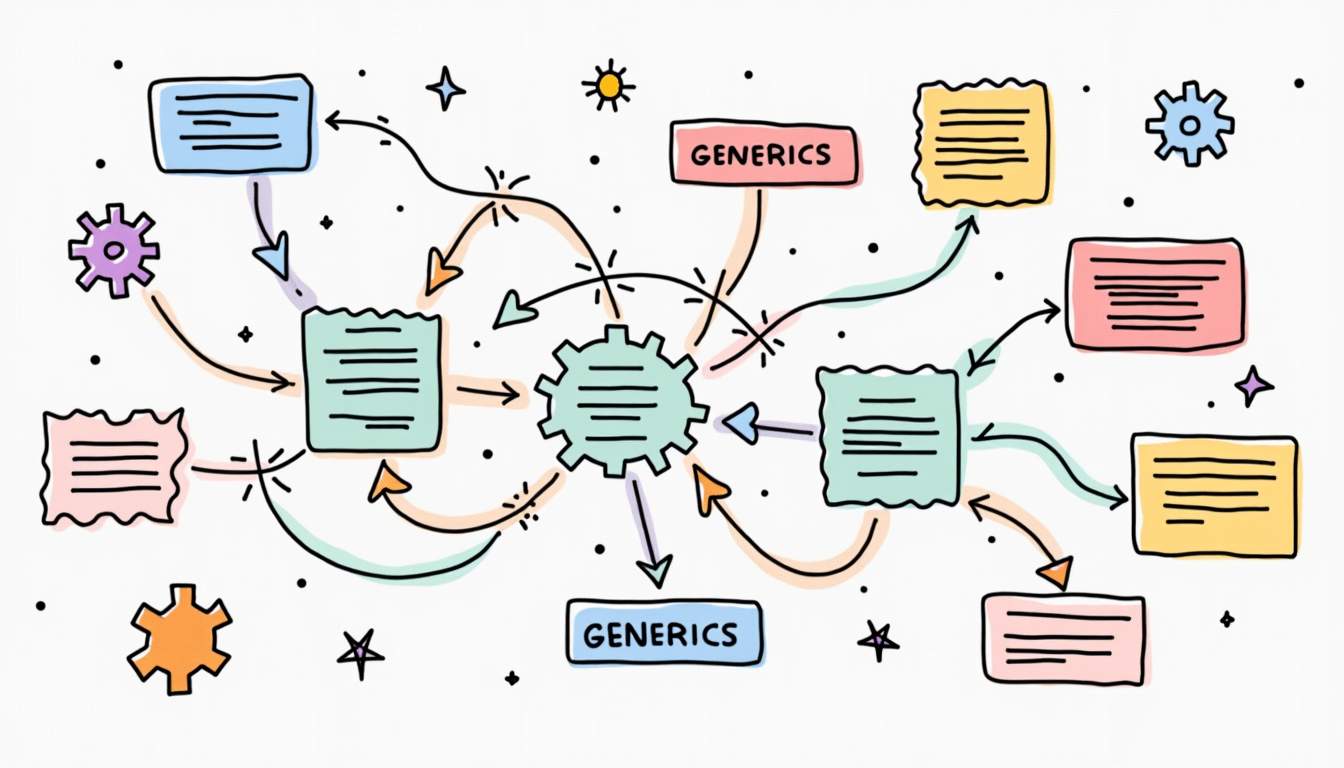
What Are Generics?
Generics provide a way to define a function or a class with a placeholder for a type. This placeholder can then be replaced with a specific type when the function or class is instantiated. For instance, a generic function can accept a parameter of any type, and TypeScript will infer the type based on the argument passed.
Here's a simple example of a generic function:
function identity(arg: T): T { return arg;}
In this example, `T` acts as a placeholder for any type. When calling the function, TypeScript will infer the type based on the argument provided, ensuring type safety throughout the function.
Benefits of Using Generics
Generics offer several advantages, including:
- Type Safety: Generics ensure that the correct data type is used, reducing runtime errors.
- Reusability: Generic components and functions can be reused with different types, promoting code efficiency.
- Flexibility: Developers can create more flexible APIs that can adapt to various data types.
Generics Constraints in TypeScript
While generics are powerful, they can become even more useful when combined with constraints. Constraints allow developers to limit the types that can be used with a generic, ensuring that only specific types are accepted.
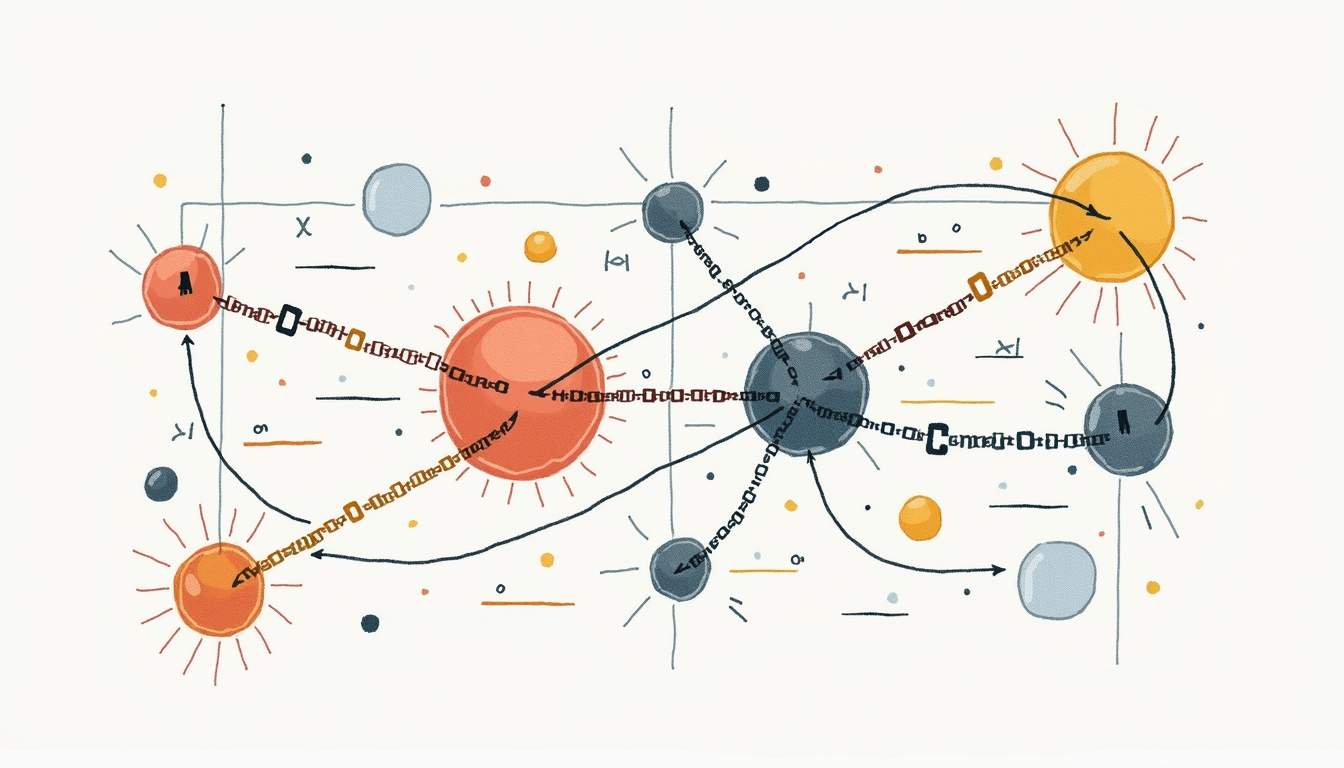
Defining Constraints
Constraints are defined using the `extends` keyword, which allows developers to specify that a generic type must extend a particular type or interface. This ensures that the generic type has certain properties or methods, making it easier to work with.
For example, consider the following code snippet:
function logLength(arg: T): void { console.log(arg.length);}
In this case, the generic type `T` is constrained to types that have a `length` property. This means that any argument passed to the `logLength` function must have a `length` property, providing additional type safety.
Using Constraints with React Hooks
When working with React hooks, generics constraints can enhance the functionality of custom hooks. By applying constraints, developers can ensure that the data types used in hooks conform to specific structures, improving code reliability.
Creating Custom Hooks with Generics Constraints
Custom hooks are a powerful feature of React, allowing developers to encapsulate logic and state management. By incorporating generics constraints into custom hooks, developers can create reusable hooks that are type-safe and adaptable to various data types.
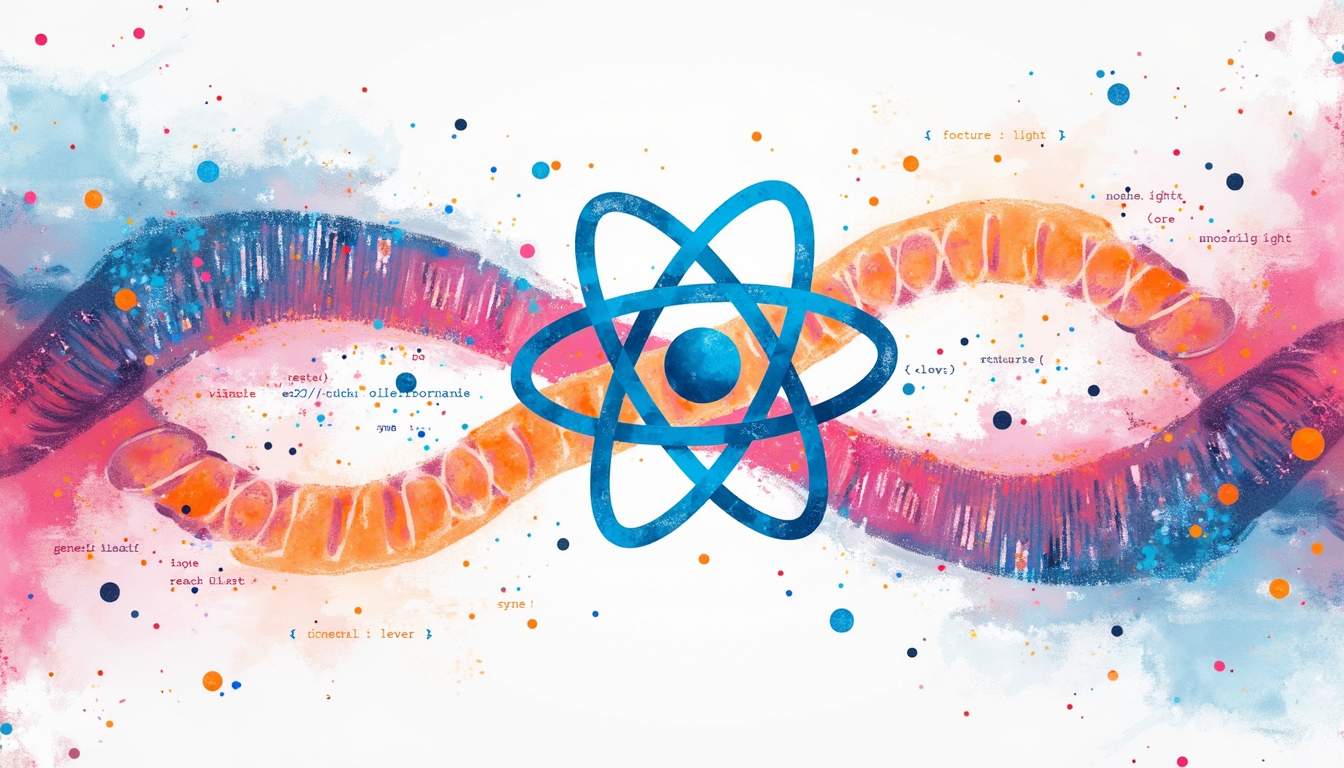
Example: A Custom Fetch Hook
Consider a scenario where a custom hook is needed to fetch data from an API. By using generics constraints, the hook can be designed to accept different data types while ensuring that the fetched data adheres to a specific structure.
import { useState, useEffect } from 'react';function useFetch(url: string): { data: T | null; error: string | null } { const [data, setData] = useState(null); const [error, setError] = useState(null); useEffect(() => { const fetchData = async () => { try { const response = await fetch(url); if (!response.ok) throw new Error('Network response was not ok'); const result: T = await response.json(); setData(result); } catch (err) { setError(err.message); } }; fetchData(); }, [url]); return { data, error };}
In this example, the `useFetch` hook is defined with a generic type `T`. This allows the hook to return data of any type specified when the hook is called. Additionally, the hook handles errors gracefully, providing a robust solution for fetching data.
Using the Custom Hook
To utilize the `useFetch` hook, developers can specify the data type they expect to receive from the API. This ensures that the data returned is type-safe and adheres to the expected structure.
interface User { id: number; name: string; email: string;}const App = () => { const { data, error } = useFetch('https://api.example.com/users'); if (error) return Error: {error}; if (!data) return Loading...; return ( {data.map(user => ( - {user.name} - {user.email}
))}
);};
In this example, the `useFetch` hook is called with the expected data type `User[]`. This ensures that the data returned from the API is an array of `User` objects, providing type safety and preventing potential runtime errors.
Advanced Generics Constraints
As developers become more comfortable with generics constraints, they can explore advanced techniques to further enhance their code. These techniques can include combining multiple constraints, using default types, and working with conditional types.
Combining Multiple Constraints
Sometimes, it may be necessary to impose multiple constraints on a generic type. This can be achieved by using the `&` operator, allowing developers to specify that a generic type must extend multiple types or interfaces.
interface Identifiable { id: number;}interface Nameable { name: string;}function logEntity(entity: T): void { console.log(`ID: ${entity.id}, Name: ${entity.name}`);}
In this example, the `logEntity` function requires a generic type `T` that extends both `Identifiable` and `Nameable`. This ensures that any argument passed to the function has both an `id` and a `name` property, enhancing type safety.
Default Types in Generics
TypeScript also allows developers to specify default types for generics. This can be particularly useful when a specific type is commonly used, allowing developers to omit the type argument when calling the function or component.
function logArray(arr: T[]): void { console.log(arr);}
In this example, the `logArray` function has a default type of `any` for the generic type `T`. This means that if no type argument is provided, TypeScript will default to `any`, allowing for flexibility while still providing type safety when a specific type is specified.
Conditional Types
Conditional types are another advanced feature of TypeScript that can be combined with generics. They allow developers to create types that depend on a condition, providing even greater flexibility in type definitions.
type IsString = T extends string ? 'Yes' : 'No';type Result1 = IsString; // 'Yes'type Result2 = IsString; // 'No'
In this example, the `IsString` type checks whether a given type `T` extends `string`. If it does, it resolves to `'Yes'`; otherwise, it resolves to `'No'`. This can be particularly useful for creating complex types based on conditions.
Best Practices for Using Generics Constraints
To effectively leverage generics constraints in TypeScript, developers should adhere to several best practices. These practices can enhance code readability, maintainability, and overall quality.
Keep It Simple
While generics constraints can be powerful, it's essential to keep them as simple as possible. Overly complex constraints can make code difficult to read and understand. Strive for clarity by using straightforward constraints that are easy to follow.
Document Your Code
When using generics constraints, thorough documentation is crucial. Clear comments and type annotations can help other developers (or your future self) understand the purpose and usage of generics in your code. Providing examples in documentation can also be beneficial.
Test Thoroughly
Generics can introduce subtle bugs if not tested properly. Ensure that all edge cases are considered and tested, especially when working with complex constraints. Writing unit tests can help catch potential issues early in the development process.
Conclusion
Mastering TypeScript generics constraints in React hooks can significantly enhance the robustness and flexibility of your applications. By understanding the fundamentals of generics, applying constraints effectively, and following best practices, developers can create reusable, type-safe components that stand the test of time.
As TypeScript continues to evolve, staying updated with the latest features and best practices will ensure that developers can harness the full power of this language in their React projects. Embrace the journey of mastering generics constraints, and watch your code quality soar.
Take Your TypeScript Projects to the Next Level with Engine Labs
Ready to supercharge your development process with the power of TypeScript and React? Engine Labs is here to elevate your software engineering workflow. By integrating with your favorite project management tools, Engine streamlines your development cycle, turning tickets into pull requests with unparalleled efficiency. Reduce your backlog and focus on crafting exceptional type-safe components with the help of Engine. Get Started today and experience the future of software engineering, making your projects more robust and flexible than ever before.