How to Resolve Rust Lifetime Errors: A Step-by-Step Guide
Rust is a powerful programming language known for its focus on safety and performance. However, one of the most common hurdles developers face when working with Rust is understanding and resolving lifetime errors. Lifetimes in Rust are a way of expressing the scope of validity of references, and they ensure that data is used safely without dangling references. This guide will walk you through the process of resolving lifetime errors step-by-step, providing you with the tools and knowledge to tackle these challenges effectively.
Understanding Rust Lifetimes
Before diving into resolving lifetime errors, it's crucial to grasp the concept of lifetimes in Rust. Lifetimes are annotations that tell the Rust compiler how long references should be valid. They are essential for ensuring memory safety without needing a garbage collector.

What Are Lifetimes?
Lifetimes are denoted using an apostrophe followed by a name, such as `'a`. They indicate the scope of a reference, ensuring that it does not outlive the data it points to. For example, if a function takes a reference to a variable, the lifetime of that reference must be shorter than or equal to the lifetime of the variable. This mechanism allows developers to express the relationships between different pieces of data in their programs, making it clear when data can be safely accessed and when it should not be used.
Moreover, lifetimes can be inferred by the Rust compiler in many cases, which simplifies the development process. However, there are situations where explicit lifetime annotations are necessary, particularly when dealing with complex data structures or when multiple references are involved. Understanding how to annotate lifetimes correctly can significantly reduce the frustration of encountering compiler errors related to invalid references, making it easier to write robust and efficient Rust code.
Why Are Lifetimes Important?
By enforcing lifetimes, Rust prevents common programming errors, such as use-after-free and dangling pointers. These errors can lead to undefined behavior in other languages, but Rust’s ownership model and lifetime system help to eliminate them at compile time. This not only enhances safety but also improves performance by allowing for more efficient memory management.
In addition to preventing memory-related bugs, lifetimes also facilitate concurrent programming. In a multi-threaded environment, ensuring that references remain valid across threads is crucial. Rust's lifetime system helps developers manage data access in a way that prevents data races, allowing for safe concurrency. By clearly defining the lifetimes of references, developers can write code that is not only safe but also takes full advantage of Rust's performance capabilities, making it a powerful tool for building reliable software systems.
Common Lifetime Errors
As you begin coding in Rust, you may encounter several types of lifetime errors. Understanding these errors is the first step in resolving them effectively. Here are some of the most common lifetime-related issues you might face:
Dangling References
A dangling reference occurs when a reference points to data that has been deallocated. This can happen if a reference is created to a local variable that goes out of scope. Rust’s borrow checker prevents this situation by ensuring that references cannot outlive their data. The borrow checker enforces strict rules at compile time, which helps developers catch these issues early in the development process, thus avoiding potential runtime errors that could lead to undefined behavior.
Conflicting Lifetimes
Another common error arises when multiple references with different lifetimes are used in a way that the compiler cannot guarantee safety. For instance, if you have a function that returns a reference, the lifetime of that reference must be clearly defined in relation to the input parameters. This can be particularly tricky when dealing with structs or complex data types that contain multiple references. Understanding how to use lifetime elision can simplify your code, but it’s essential to grasp the underlying principles to avoid conflicts that could compromise the integrity of your program.
Missing Lifetime Annotations
Sometimes, the compiler may require explicit lifetime annotations to understand how different references relate to each other. Failing to provide these annotations can lead to compilation errors. Understanding when and how to annotate lifetimes is crucial for writing safe and efficient Rust code. It’s also worth noting that while the Rust compiler is quite adept at inferring lifetimes in many cases, there are scenarios—especially in more complex functions—where explicit annotations are necessary to guide the compiler. This not only helps with compilation but also serves as documentation for other developers who may read your code later.
In addition to these common errors, it’s important to familiarize yourself with Rust's lifetime elision rules, which can often reduce the need for explicit annotations in straightforward cases. These rules allow the compiler to infer lifetimes based on common patterns, making your code cleaner and more readable. However, relying solely on elision can sometimes lead to confusion, especially for newcomers. Therefore, a solid understanding of how lifetimes work will empower you to write more robust code and troubleshoot issues more effectively as you progress in your Rust programming journey.
Step-by-Step Guide to Resolving Lifetime Errors
Now that the basics are clear, let’s explore a systematic approach to resolving lifetime errors in Rust. This guide will provide practical steps and examples to illustrate each point.
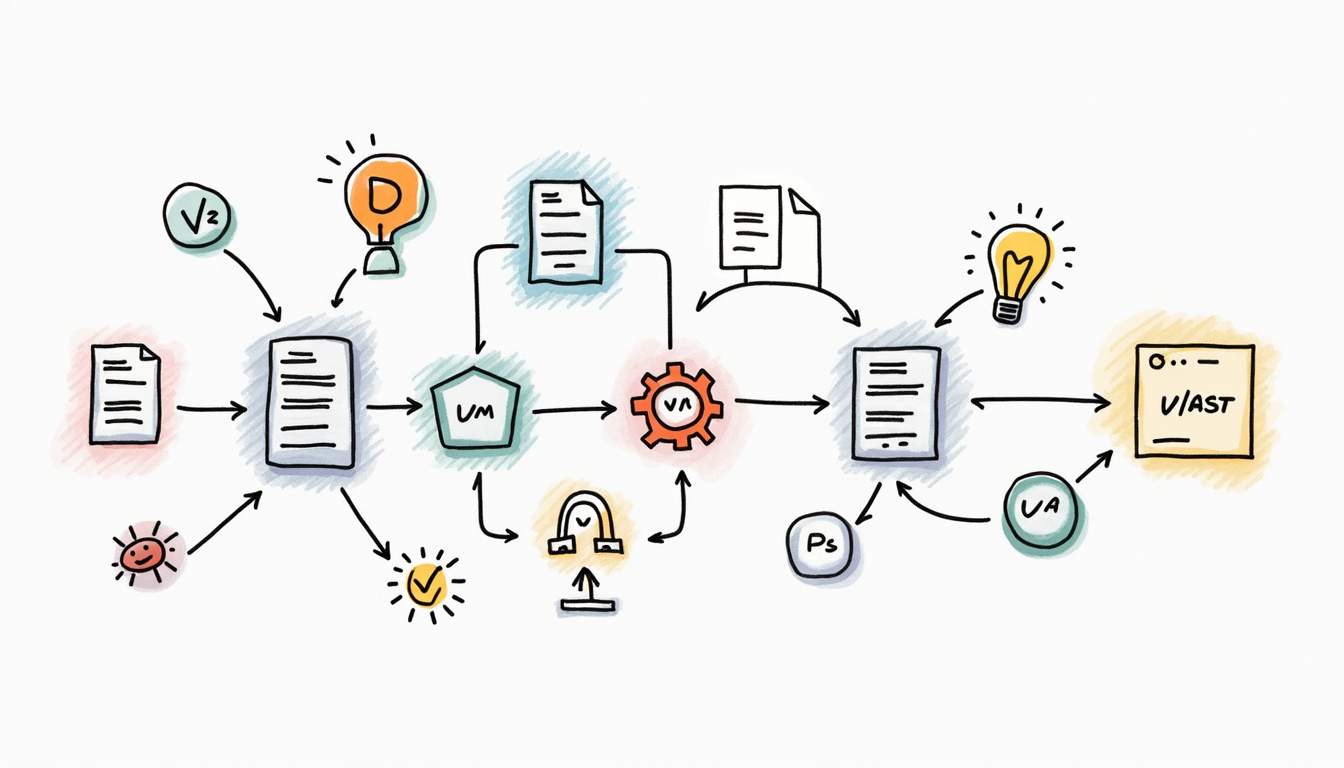
Step 1: Identify the Error
The first step in resolving any lifetime error is to carefully read the error message provided by the Rust compiler. Rust’s error messages are often detailed and can point you directly to the issue. Pay attention to the specific references and lifetimes mentioned in the error message.
Step 2: Analyze the Code
Once the error is identified, analyze the code to understand the relationships between the references involved. Look for any potential dangling references, conflicting lifetimes, or missing annotations. It can be helpful to draw a diagram or write out the lifetimes to visualize how they interact.
Step 3: Add Lifetime Annotations
If the compiler indicates that lifetime annotations are needed, add them to your function signatures or struct definitions. For example, if a function takes two references and returns one, you might need to specify the lifetimes like this:
fn longest<'a>(s1: &'a str, s2: &'a str) -> &'a str { ... }
This annotation indicates that the returned reference will have the same lifetime as the shortest of the two input references.
Practical Examples of Resolving Lifetime Errors
To solidify understanding, let’s look at some practical examples of common lifetime errors and how to resolve them.
Example 1: Dangling Reference
Consider the following code snippet that attempts to return a reference to a local variable:
fn dangle() -> &String { let s = String::from("hello"); &s}
This code will result in a dangling reference error because `s` goes out of scope at the end of the function. To resolve this, you can return the ownership of the string instead:
fn no_dangle() -> String { let s = String::from("hello"); s}
In this case, the function returns the owned `String`, ensuring that there are no dangling references.
Example 2: Conflicting Lifetimes
Another common scenario involves conflicting lifetimes. Consider the following function:
fn first_word(s: &String) -> &str { let bytes = s.as_bytes(); for (i, &item) in bytes.iter().enumerate() { if item == b' ' { return &s[0..i]; } } &s[..]}
This function attempts to return a slice of the string, but it will fail due to conflicting lifetimes. The solution is to adjust the function signature to include lifetimes:
fn first_word<'a>(s: &'a String) -> &'a str { ... }
This annotation clarifies that the returned slice will have the same lifetime as the input string reference.
Example 3: Missing Lifetime Annotations
Sometimes, the compiler requires explicit lifetime annotations that are not immediately obvious. For instance, consider this code:
fn longest<'a>(s1: &'a str, s2: &str) -> &'a str { ... }
The function signature is missing a lifetime for `s2`. To fix this, you can add a lifetime annotation to `s2` as well:
fn longest<'a, 'b>(s1: &'a str, s2: &'b str) -> &'a str { ... }
This change ensures that both references are correctly annotated, allowing the compiler to understand their relationships.
Advanced Lifetime Concepts
Once you have a solid grasp of the basics, it’s beneficial to explore some advanced lifetime concepts that can further enhance your understanding and coding skills.
Lifetime Bounds
Lifetime bounds allow you to specify relationships between lifetimes in more complex scenarios. For instance, you can use lifetime bounds to indicate that one lifetime must outlive another. This is particularly useful in generic structs and traits.
struct Wrapper<'a> { value: &'a str,}
In this example, the `Wrapper` struct holds a reference with a lifetime `'a`, ensuring that the reference remains valid as long as the `Wrapper` instance exists.
Static Lifetimes
The `'static` lifetime is a special lifetime that signifies that the data is available for the entire duration of the program. This is often used for string literals or data that is stored in the binary. Understanding when to use `'static` can help prevent lifetime errors in certain contexts.
fn static_str() -> &'static str { "I live for the entire program!"}
This function returns a string literal with a `'static` lifetime, ensuring that it remains valid throughout the program's execution.
Lifetime Elision
Rust also supports lifetime elision, which allows the compiler to infer lifetimes in certain situations, reducing the need for explicit annotations. For example, in functions that take one input reference and return a reference, the compiler can automatically infer the lifetimes:
fn first<'a>(s: &'a str) -> &'a str { s }
Here, the compiler understands that the returned reference has the same lifetime as the input reference, allowing for cleaner code.
Conclusion
Resolving lifetime errors in Rust can be challenging, but with a solid understanding of lifetimes and a systematic approach, it becomes manageable. By identifying errors, analyzing code, and adding the necessary annotations, developers can effectively navigate the complexities of Rust's ownership model.

As you continue to work with Rust, remember that practice is key. The more you engage with lifetimes and their intricacies, the more intuitive they will become. With this guide, you now have the tools and knowledge to tackle lifetime errors confidently, paving the way for safer and more efficient Rust programming.
Take Your Rust Projects to the Next Level with Engine Labs
As you refine your skills in resolving Rust lifetime errors, why not enhance your development workflow with Engine Labs? Engine is the AI-driven software engineer that integrates with your favorite project management tools, turning tickets into pull requests with unparalleled efficiency. Reduce your backlog and supercharge your development cycle, allowing you to focus on crafting safe and efficient Rust code. Ready to revolutionize the way you build software? Get Started with Engine Labs today and experience the future of software engineering.